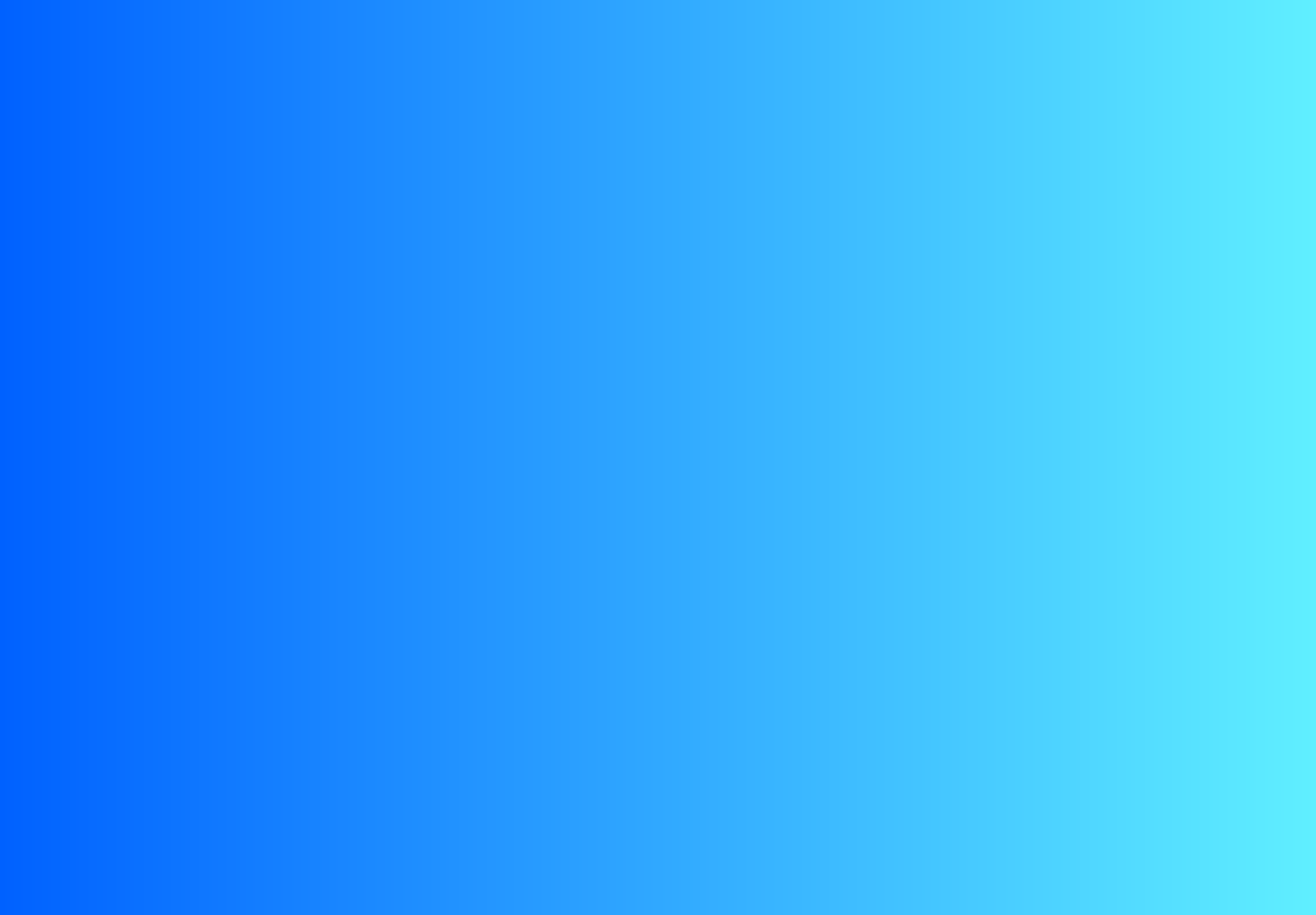
CORS Headers
CORS Headers: A Comprehensive Guide
Cross-Origin Resource Sharing (CORS) is a security feature implemented by browsers to prevent web pages from making requests to a different domain than the one that served the web page. CORS headers are HTTP headers that the server includes in its responses to allow or restrict cross-origin requests. This guide will explain the key CORS headers, how they work, and how to use them effectively.
Access-Control-Allow-Origin
This header specifies which origins are allowed to access the resource. It can have one of the following values:
- A specific origin (e.g.,
https://example.com
). *
(to allow all origins).
Example:
Access-Control-Allow-Origin: https://example.com
Access-Control-Allow-Methods
This header specifies which HTTP methods are allowed for cross-origin requests. Common methods include GET
, POST
, PUT
, DELETE
, and OPTIONS
.
Example:
Access-Control-Allow-Methods: GET, POST, PUT, DELETE
Access-Control-Allow-Headers
This header specifies which headers are allowed in the actual request. Common headers include Authorization
, Content-Type
, and X-Requested-With
.
Example:
Access-Control-Allow-Headers: Authorization, Content-Type
Access-Control-Allow-Credentials
This header indicates whether credentials (e.g., cookies, authorization headers) can be included in the request. It can have one of the following values:
true
(to allow credentials).false
(to disallow credentials).
Example:
Access-Control-Allow-Credentials: true
Access-Control-Expose-Headers
This header specifies which headers can be exposed to the client. By default, only simple response headers (e.g., Cache-Control
, Content-Language
, Content-Type
, Expires
, Last-Modified
, Pragma
) are exposed.
Example:
Access-Control-Expose-Headers: X-Custom-Header
Access-Control-Max-Age
This header specifies how long the results of a preflight request can be cached (in seconds).
Example:
Access-Control-Max-Age: 3600
How CORS Headers Work
Simple Requests
For simple requests (e.g., GET
or POST
with certain content types), the browser sends the request directly to the server. The server responds with the appropriate CORS headers, and the browser checks if the Access-Control-Allow-Origin
header matches the request origin.
Example:
- Request:
GET /api/data HTTP/1.1 Host: backend.com Origin: https://frontend.com
- Response:
HTTP/1.1 200 OK Access-Control-Allow-Origin: https://frontend.com Content-Type: application/json {"message": "Hello, CORS is enabled!"}
Preflight Requests
For complex requests (e.g., PUT
, DELETE
, or requests with custom headers), the browser sends a preflight request (OPTIONS
) to the server. The server responds with the appropriate CORS headers, and the browser checks if the actual request is allowed.
Example:
- Preflight Request:
OPTIONS /api/data HTTP/1.1 Host: backend.com Origin: https://frontend.com Access-Control-Request-Method: PUT Access-Control-Request-Headers: Authorization, Content-Type
- Preflight Response:
HTTP/1.1 204 No Content Access-Control-Allow-Origin: https://frontend.com Access-Control-Allow-Methods: GET, POST, PUT, DELETE Access-Control-Allow-Headers: Authorization, Content-Type Access-Control-Max-Age: 3600
Common Issues and Solutions
1. CORS Error: No Access-Control-Allow-Origin Header
This error occurs when the server does not include the Access-Control-Allow-Origin
header in its response. Ensure that the server is configured to include this header. Here is a guide on how to fix this issue?
2. CORS Error: Preflight Request Failed
This error occurs when the server does not handle preflight requests correctly. Ensure that the server responds to OPTIONS
requests with the appropriate CORS headers.
3. CORS Error: Invalid Access-Control-Allow-Origin Value
This error occurs when the Access-Control-Allow-Origin
header has an invalid value. Ensure that the header contains a valid origin or *
.
Best Practices for Using CORS Headers
- Restrict Allowed Origins: Avoid using
*
forAccess-Control-Allow-Origin
in production. Instead, specify the exact origins that are allowed. - Use Secure Methods and Headers: Only allow necessary HTTP methods and headers.
- Allow Credentials Only When Necessary: Use
Access-Control-Allow-Credentials: true
only if your application requires cookies or authentication tokens. - Test Thoroughly: Use browser developer tools or API testing tools to verify that CORS headers are correctly set.
Real-World Example: Frontend-Backend Communication
Scenario:
You have a frontend application hosted on https://frontend.com
that needs to fetch data from a backend API hosted on https://backend.com
.
Solution:
- Configure the backend to include the following CORS headers in its responses:
Access-Control-Allow-Origin: https://frontend.com Access-Control-Allow-Methods: GET, POST, PUT, DELETE Access-Control-Allow-Headers: Authorization, Content-Type Access-Control-Allow-Credentials: true
- Ensure the frontend uses the correct API endpoint:
fetch('https://backend.com/api/data', { headers: { 'Authorization': 'Bearer token', 'Content-Type': 'application/json' } }) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
Conclusion
CORS headers play a crucial role in enabling secure cross-origin requests while maintaining web security. Properly configuring headers like Access-Control-Allow-Origin
, Access-Control-Allow-Methods
, and Access-Control-Allow-Headers
ensures seamless communication between origins without exposing applications to security risks. Understanding and implementing these headers correctly helps developers build more secure and flexible web applications.