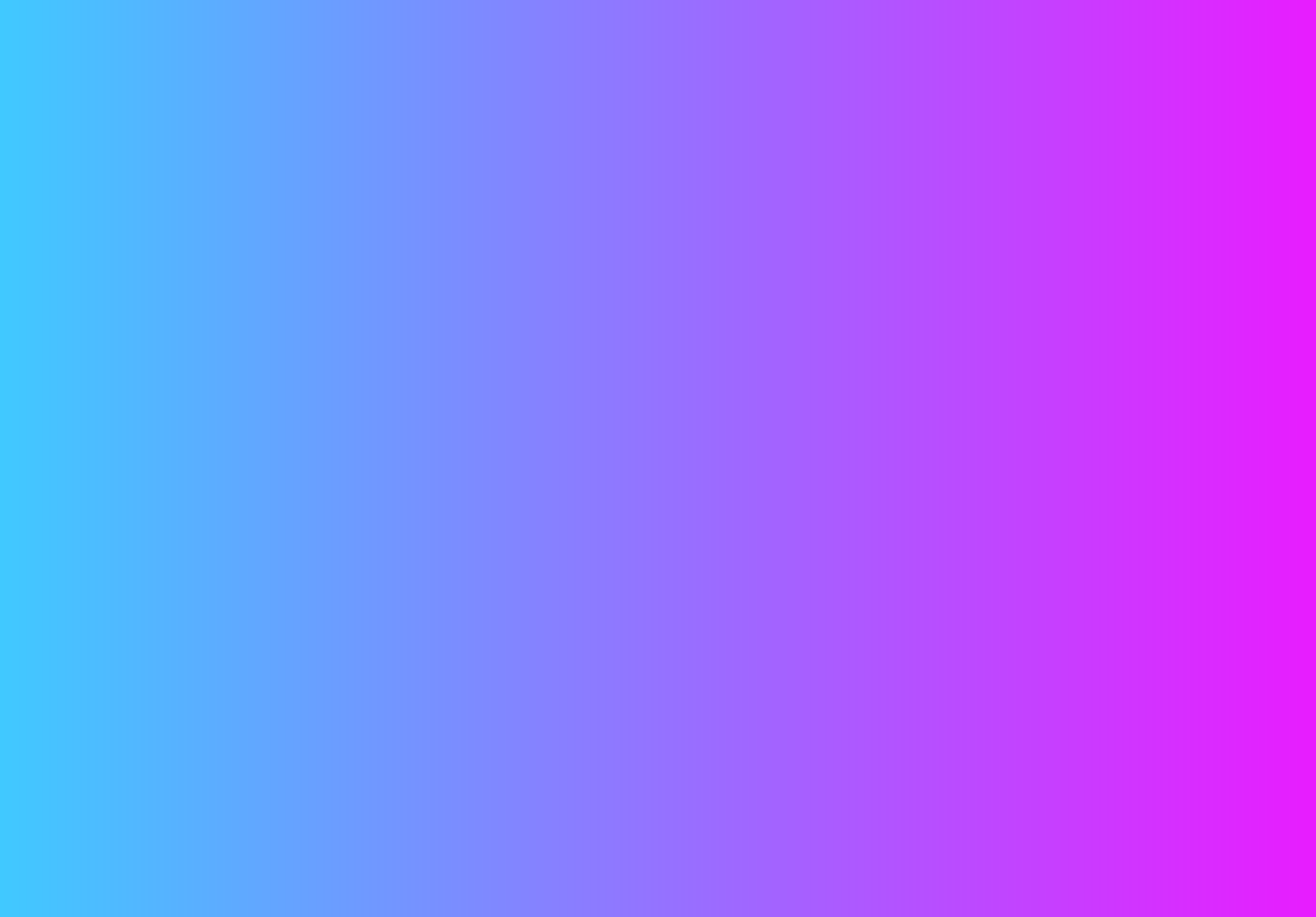
Same Origin Policy
Same-Origin Policy: A Comprehensive Guide
The Same-Origin Policy (SOP) is a critical security mechanism implemented by web browsers to prevent web pages from making requests to a different domain than the one that served the web page. This policy is essential for protecting users from malicious attacks, such as Cross-Site Request Forgery (CSRF) and data theft. In this guide, we’ll explore what the Same-Origin Policy is, how it works, and its implications for web development.
What is the Same-Origin Policy?
The Same-Origin Policy restricts how a document or script loaded from one origin can interact with resources from another origin. An origin is defined by the combination of:
- Protocol (e.g.,
http
,https
) - Host (e.g.,
example.com
) - Port (e.g.,
80
,443
)
Two URLs are considered to have the same origin if their protocol, host, and port are identical. For example:
https://example.com
andhttps://example.com/api
have the same origin.https://example.com
andhttp://example.com
have different origins (different protocols).https://example.com
andhttps://api.example.com
have different origins (different hosts).
How Does the Same-Origin Policy Work?
The Same-Origin Policy applies to:
- AJAX Requests: Browsers block cross-origin
XMLHttpRequest
orfetch
requests unless the server explicitly allows them using CORS headers. - DOM Access: Scripts running on one origin cannot access the DOM of a page from another origin.
- Cookies and Storage: Cookies and web storage (e.g.,
localStorage
,sessionStorage
) are isolated by origin.
Example: Same-Origin vs. Cross-Origin Requests
Same-Origin Request:
- Frontend URL:
https://example.com
- API URL:
https://example.com/api/data
- Result: The browser allows the request because the protocol, host, and port match.
Cross-Origin Request:
- Frontend URL:
https://frontend.com
- API URL:
https://backend.com/api/data
- Result: The browser blocks the request unless the backend includes the appropriate CORS headers.
Why is the Same-Origin Policy Important?
The Same-Origin Policy is crucial for:
- Preventing Data Theft: It stops malicious websites from accessing sensitive data from other origins (e.g., banking sessions).
- Mitigating CSRF Attacks: It prevents attackers from making unauthorized requests on behalf of a user.
- Isolating Web Applications: It ensures that web applications from different origins cannot interfere with each other.
Exceptions to the Same-Origin Policy
While the Same-Origin Policy is strict, there are some exceptions:
-
Cross-Origin Resource Sharing (CORS):
- Servers can allow cross-origin requests by including specific headers (e.g.,
Access-Control-Allow-Origin
). - Example:
Access-Control-Allow-Origin: https://frontend.com
- Servers can allow cross-origin requests by including specific headers (e.g.,
-
JSONP (JSON with Padding):
- A legacy technique for making cross-origin requests using
<script>
tags. - Example:
<script src="https://backend.com/api/data?callback=handleData"></script>
- A legacy technique for making cross-origin requests using
-
Cross-Origin Embedding:
- Browsers allow embedding resources (e.g., images, scripts, iframes) from different origins.
- Example:
<img src="https://example.com/image.jpg" alt="Cross-Origin Image">
-
PostMessage API:
- Allows secure communication between windows or iframes from different origins.
- Example:
// Sending a message window.postMessage("Hello", "https://example.com"); // Receiving a message window.addEventListener("message", (event) => { if (event.origin === "https://example.com") { console.log(event.data); // "Hello" } });
Implications for Web Development
1. Cross-Origin API Requests
If your frontend and backend are hosted on different origins, you need to configure CORS on the server to allow cross-origin requests. For example:
- In Node.js (Express):
app.use((req, res, next) => { res.header('Access-Control-Allow-Origin', 'https://frontend.com'); res.header('Access-Control-Allow-Methods', 'GET, POST, PUT, DELETE'); res.header('Access-Control-Allow-Headers', 'Content-Type, Authorization'); next(); });
2. Cross-Origin Embedding
You can embed resources (e.g., images, scripts, iframes) from different origins without issues. However, accessing the content of cross-origin iframes is restricted unless the postMessage
API is used.
3. Cookies and Storage
Cookies and web storage are isolated by origin. If you need to share data across subdomains, you can configure cookies with the Domain
attribute:
Set-Cookie: sessionId=abc123; Domain=.example.com; Path=/
Real-World Example: Frontend-Backend Communication
Scenario:
You have a frontend application hosted on https://frontend.com
that needs to fetch data from a backend API hosted on https://backend.com
.
Solution:
- Configure the backend to allow cross-origin requests from
https://frontend.com
using CORS headers:Access-Control-Allow-Origin: https://frontend.com Access-Control-Allow-Methods: GET, POST, PUT, DELETE Access-Control-Allow-Headers: Content-Type, Authorization
- Ensure the frontend uses the correct API endpoint:
fetch('https://backend.com/api/data', { headers: { 'Authorization': 'Bearer token', 'Content-Type': 'application/json' } }) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
Best Practices for Working with the Same-Origin Policy
- Use CORS for Cross-Origin Requests: Configure your server to allow cross-origin requests from trusted origins.
- Avoid JSONP: JSONP is outdated and insecure. Use CORS instead.
- Secure Cross-Origin Communication: Use the
postMessage
API for secure communication between windows or iframes. - Isolate Sensitive Data: Use the Same-Origin Policy to protect sensitive data from unauthorized access.
Conclusion
The Same-Origin Policy (SOP) is a key security feature that prevents unauthorized cross-origin access, protecting user data. While it enhances security, it also restricts legitimate interactions, leading to the use of CORS for controlled access. Developers must configure CORS carefully to balance security and functionality, ensuring safe cross-origin communication.