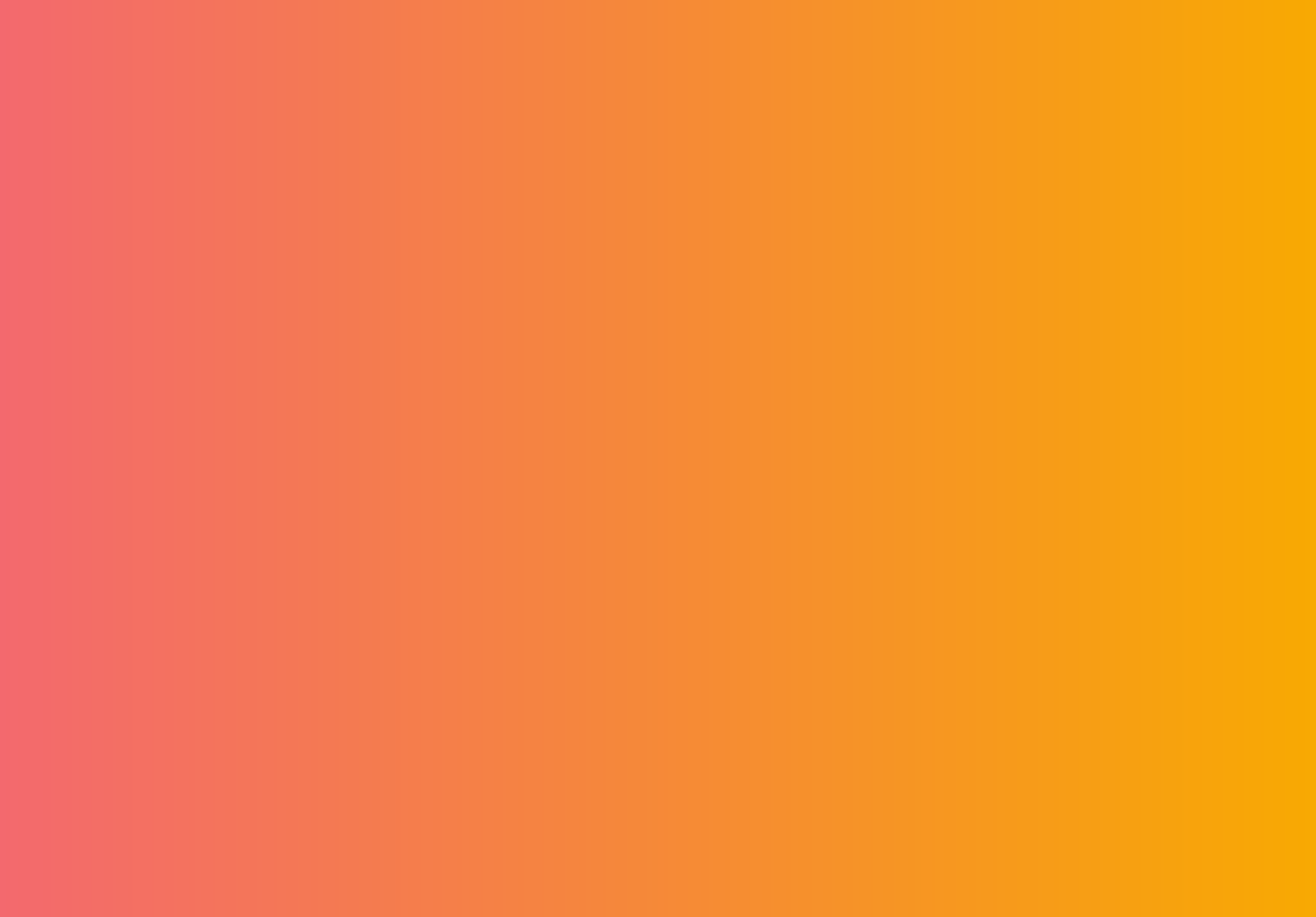
CORS for Developers - Django
CORS for Developers: Django
Cross-Origin Resource Sharing (CORS) is a security feature implemented by browsers to prevent web pages from making requests to a different domain than the one that served the web page. If you’re developing a Django application that serves APIs to a frontend hosted on a different domain, you need to configure CORS properly. This guide will walk you through how to handle CORS in Django, including common issues and best practices.
Why CORS Matters in Django
When your Django backend and frontend are hosted on different domains (e.g., https://backend.com
and https://frontend.com
), the browser will block requests from the frontend to the backend unless the backend explicitly allows it. This is where CORS comes into play. Django, by default, does not include CORS headers in its responses, so you need to configure it manually.
How to Enable CORS in Django
1. Install django-cors-headers
The easiest way to handle CORS in Django is to use the django-cors-headers
package. This package provides middleware to add the necessary CORS headers to your responses.
Install the package using pip:
pip install django-cors-headers
2. Add corsheaders
to Your Installed Apps
In your Django project’s settings.py
file, add corsheaders
to the INSTALLED_APPS
list:
INSTALLED_APPS = [
...
'corsheaders',
...
]
3. Add CORS Middleware
Add the CorsMiddleware
to your middleware stack. Make sure it is placed before CommonMiddleware
or any other middleware that generates responses:
MIDDLEWARE = [
...
'corsheaders.middleware.CorsMiddleware',
'django.middleware.common.CommonMiddleware',
...
]
4. Configure CORS Settings
In your settings.py
file, configure the CORS settings to allow specific origins, methods, and headers.
Allow All Origins (Not Recommended for Production)
To allow requests from all origins, add the following:
CORS_ALLOW_ALL_ORIGINS = True
Allow Specific Origins (Recommended)
To allow requests only from specific origins, use the CORS_ALLOWED_ORIGINS
setting:
CORS_ALLOWED_ORIGINS = [
"https://frontend.com",
"https://example.com",
]
Allow Specific Methods and Headers
You can also specify which HTTP methods and headers are allowed:
CORS_ALLOW_METHODS = [
'GET',
'POST',
'PUT',
'PATCH',
'DELETE',
'OPTIONS',
]
CORS_ALLOW_HEADERS = [
'Authorization',
'Content-Type',
]
5. Handle Preflight Requests
Preflight requests are OPTIONS
requests sent by the browser to check if the server allows the actual request. The django-cors-headers
package automatically handles preflight requests, so you don’t need to do anything extra.
Example: Complete Django CORS Configuration
Here’s a complete example of a settings.py
file with CORS configured:
INSTALLED_APPS = [
...
'corsheaders',
...
]
MIDDLEWARE = [
...
'corsheaders.middleware.CorsMiddleware',
'django.middleware.common.CommonMiddleware',
...
]
# Allow specific origins
CORS_ALLOWED_ORIGINS = [
"https://frontend.com",
"https://example.com",
]
# Allow specific methods
CORS_ALLOW_METHODS = [
'GET',
'POST',
'PUT',
'PATCH',
'DELETE',
'OPTIONS',
]
# Allow specific headers
CORS_ALLOW_HEADERS = [
'Authorization',
'Content-Type',
]
Common Issues and Solutions
1. CORS Error: No Access-Control-Allow-Origin
Header
This error occurs when the server does not include the Access-Control-Allow-Origin
header in its response. Ensure that:
django-cors-headers
is installed and configured correctly.- The
CorsMiddleware
is placed beforeCommonMiddleware
.
2. CORS Error: Preflight Request Failed
This error occurs when the server does not handle OPTIONS
requests correctly. The django-cors-headers
package automatically handles preflight requests, so ensure it is properly installed and configured.
3. CORS Error: Invalid Access-Control-Allow-Origin
Value
This error occurs when the Access-Control-Allow-Origin
header has an invalid value. Ensure that the CORS_ALLOWED_ORIGINS
setting contains valid origins.
Best Practices for CORS in Django
- Restrict Allowed Origins: Avoid using
CORS_ALLOW_ALL_ORIGINS
in production. Instead, specify the exact origins that are allowed. - Use Secure Methods and Headers: Only allow necessary HTTP methods and headers.
- Test Thoroughly: Use browser developer tools or API testing tools to verify that CORS headers are correctly set. You should verify the exposed API endpoint using CORS-Tester.
- Keep Middleware Order in Mind: Ensure
CorsMiddleware
is placed before any middleware that generates responses.
Real-World Example: Frontend-Backend Communication
Scenario:
You have a frontend application hosted on https://frontend.com
that needs to fetch data from a Django backend hosted on https://backend.com
.
Solution:
- Install and configure
django-cors-headers
in your Django project. - Allow requests from
https://frontend.com
by adding it toCORS_ALLOWED_ORIGINS
. - Ensure the frontend uses the correct API endpoint:
fetch('https://backend.com/api/data', { headers: { 'Authorization': 'Bearer token', 'Content-Type': 'application/json' } }) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
Conclusion
In conclusion, implementing CORS in Django is essential for ensuring that your web application can interact with external resources securely and efficiently. By configuring the appropriate headers and utilizing libraries like django-cors-headers
, developers can easily manage cross-origin requests. It’s crucial to set strict rules for allowed origins, methods, and headers to maintain security while allowing necessary cross-origin interactions. Proper CORS configuration enhances the flexibility of your Django applications while safeguarding against potential vulnerabilities.