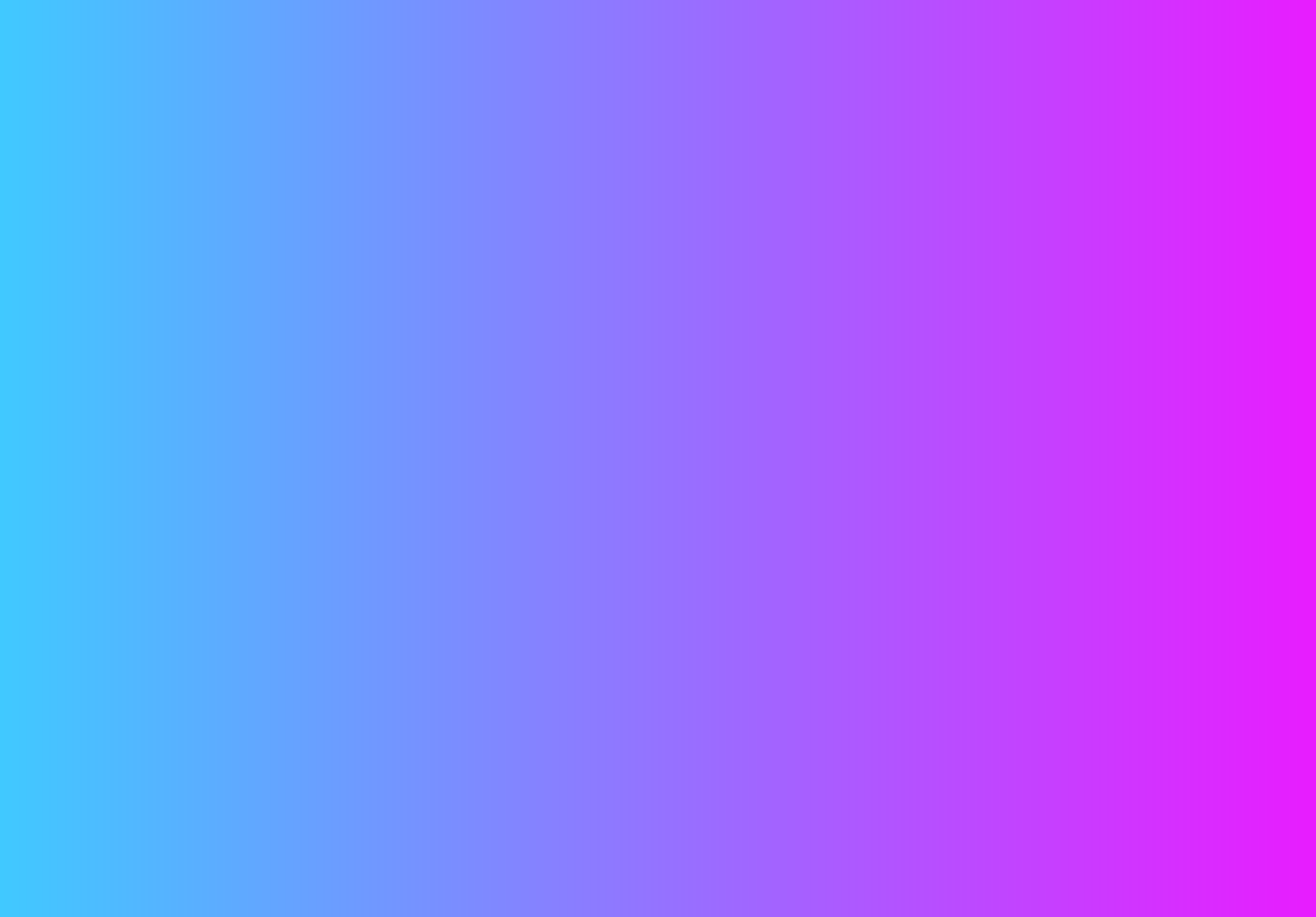
CORS for Developers - SpringBoot
CORS for Developers: Spring Boot
Cross-Origin Resource Sharing (CORS) is a security feature implemented by browsers to prevent web pages from making requests to a different domain than the one that served the web page. If you’re developing a Spring Boot application that serves APIs to a frontend hosted on a different domain, you need to configure CORS properly. This guide will walk you through how to handle CORS in Spring Boot, including common issues and best practices.
Why CORS Matters in Spring Boot
When your Spring Boot backend and frontend are hosted on different domains (e.g., https://backend.com
and https://frontend.com
), the browser will block requests from the frontend to the backend unless the backend explicitly allows it. This is where CORS comes into play. Spring Boot provides several ways to configure CORS, making it easy to enable cross-origin requests.
How to Enable CORS in Spring Boot
1. Global CORS Configuration
You can configure CORS globally for your entire Spring Boot application by defining a CorsConfigurationSource
bean.
Example:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class CorsConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/api/**")
.allowedOrigins("https://frontend.com", "https://example.com")
.allowedMethods("GET", "POST", "PUT", "DELETE", "OPTIONS")
.allowedHeaders("Authorization", "Content-Type")
.allowCredentials(true)
.maxAge(3600);
}
}
In this example:
addMapping("/api/**")
: Specifies the URL patterns to which CORS applies.allowedOrigins("https://frontend.com", "https://example.com")
: Allows requests from specific origins.allowedMethods("GET", "POST", "PUT", "DELETE", "OPTIONS")
: Specifies the allowed HTTP methods.allowedHeaders("Authorization", "Content-Type")
: Specifies the allowed headers.allowCredentials(true)
: Allows credentials (e.g., cookies) to be included in the request.maxAge(3600)
: Specifies how long the results of a preflight request can be cached (in seconds).
2. Controller-Level CORS Configuration
You can also configure CORS at the controller or method level using the @CrossOrigin
annotation.
Example:
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/api")
public class ApiController {
@CrossOrigin(origins = "https://frontend.com")
@GetMapping("/data")
public String getData() {
return "Hello, CORS is enabled!";
}
}
In this example:
- The
@CrossOrigin
annotation allows requests fromhttps://frontend.com
to the/api/data
endpoint.
3. Handling Preflight Requests
Preflight requests are OPTIONS
requests sent by the browser to check if the server allows the actual request. Spring Boot automatically handles preflight requests when CORS is configured, so you don’t need to do anything extra.
Common Issues and Solutions
1. CORS Error: No Access-Control-Allow-Origin
Header
This error occurs when the server does not include the Access-Control-Allow-Origin
header in its response. Ensure that:
- CORS is properly configured in your Spring Boot application.
- The
allowedOrigins
setting includes the origin of the frontend.
2. CORS Error: Preflight Request Failed
This error occurs when the server does not handle OPTIONS
requests correctly. Spring Boot automatically handles preflight requests when CORS is configured, so ensure your CORS configuration is correct.
3. CORS Error: Invalid Access-Control-Allow-Origin
Value
This error occurs when the Access-Control-Allow-Origin
header has an invalid value. Ensure that the allowedOrigins
setting contains valid origins.
Best Practices for CORS in Spring Boot
- Restrict Allowed Origins: Avoid using
*
forallowedOrigins
in production. Instead, specify the exact origins that are allowed. - Use Secure Methods and Headers: Only allow necessary HTTP methods and headers.
- Allow Credentials Only When Necessary: Use
allowCredentials(true)
only if your application requires cookies or authentication tokens. - Test Thoroughly: Use browser based developer tools or API testing tools to verify that CORS headers are correctly set.
Real-World Example: Frontend-Backend Communication
Scenario:
You have a frontend application hosted on https://frontend.com
that needs to fetch data from a Spring Boot backend hosted on https://backend.com
.
Solution:
- Configure CORS in your Spring Boot application to allow requests from
https://frontend.com
. - Ensure the frontend uses the correct API endpoint:
fetch('https://backend.com/api/data', { headers: { 'Authorization': 'Bearer token', 'Content-Type': 'application/json' } }) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
Conclusion
In conclusion, configuring CORS in Spring Boot is vital for enabling secure cross-origin requests between your backend and frontend applications. By using Spring’s built-in support or libraries like @CrossOrigin
, developers can easily define the allowed origins, methods, and headers for their API endpoints. Ensuring that CORS is properly configured helps maintain security while allowing your Spring Boot application to interact seamlessly with external services and clients. Proper management of CORS settings ensures both flexibility and protection for your application’s interactions.