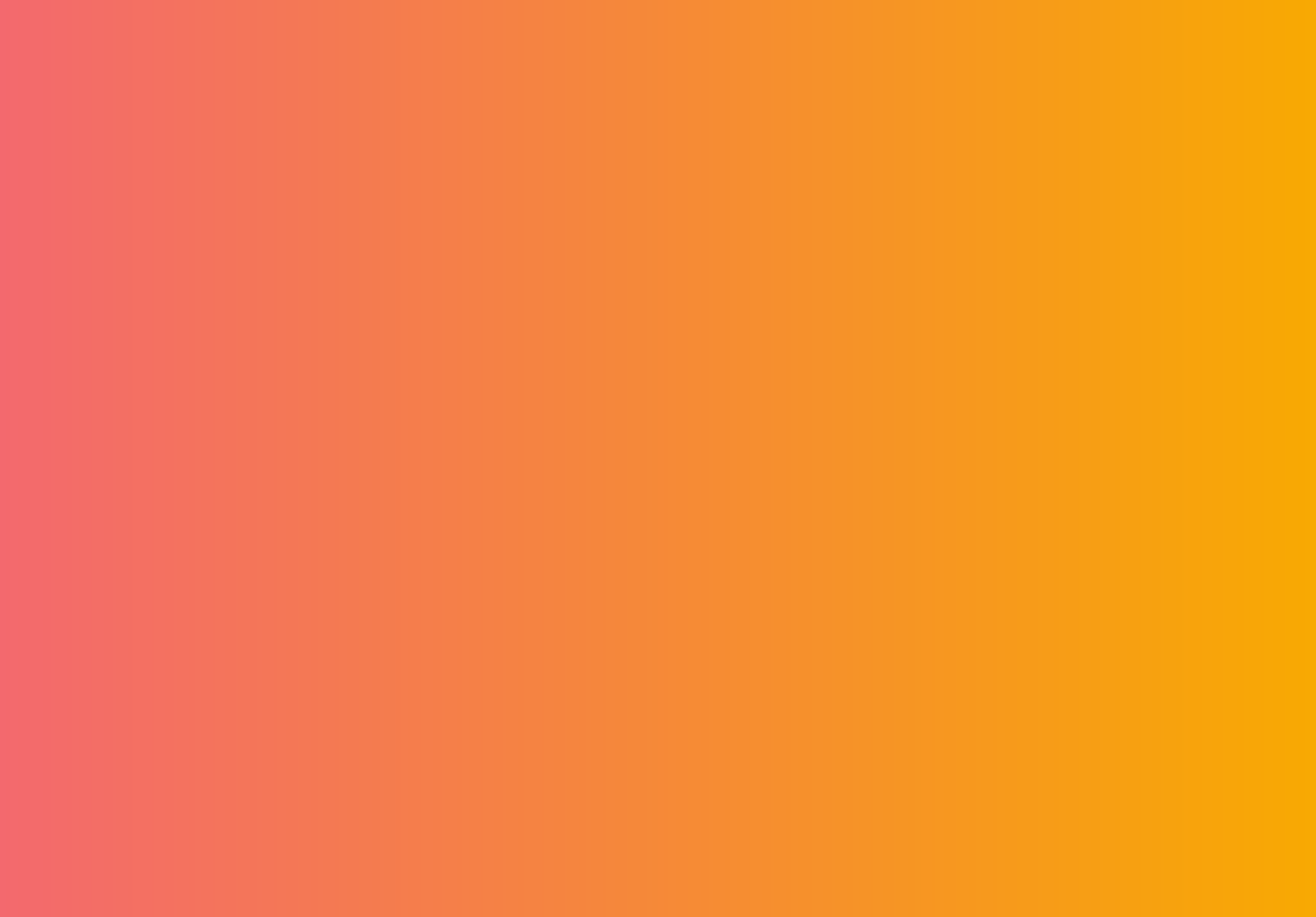
CORS Policy Blocks Redirects
Understanding CORS and Redirects
How CORS Handles Redirects
When a browser makes a cross-origin request to a URL that responds with a redirect (3xx status), it follows the redirection automatically. However, CORS policies require that both the original and redirected responses include proper CORS headers.
Why CORS Blocks Cross-Origin Redirects
If a redirected response does not have the Access-Control-Allow-Origin
header, the browser blocks it and throws a CORS error:
Access to fetch at 'https://api.example.com/data' from origin 'https://your-website.com'
has been blocked by CORS policy: Redirect is not allowed for a preflight request.
Common Scenarios Where Redirects Cause Issues
1. Redirecting from an API Request
If your API returns a 301/302 redirect to another domain, the browser will block the request unless the new location also includes proper CORS headers.
2. Redirects in OAuth Authentication Flows
OAuth flows often involve redirections between multiple domains. If one of these redirects does not have CORS headers, authentication may fail.
3. Fetch API and Automatic Redirection
By default, the Fetch API follows redirects automatically, but CORS restrictions can block them.
Example:
fetch("https://api.example.com/login", {
method: "POST",
credentials: "include"
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
If the response includes a Location
header pointing to another origin, CORS restrictions will apply.
How Browsers Handle Cross-Origin Redirects
Same-Origin vs Cross-Origin Redirects
- Same-Origin Redirects: Allowed without any CORS issues.
- Cross-Origin Redirects: The new location must include CORS headers, or the browser blocks it.
Setting redirect: “manual” in Fetch API
By default fetch API handles the redirects automatically, following the redirect by itself. To handle redirects manually, you can use:
fetch("https://api.example.com/data", {
method: "GET",
redirect: "manual"
})
.then(response => console.log(response.status))
.catch(error => console.error(error));
Setting redirect: "manual"
prevents automatic redirection, allowing you to inspect the redirect response.
Fixing CORS Issues with Redirects
1. Handling Redirects in the Frontend
If you control the frontend, avoid automatic redirects and instead manually handle them:
fetch("https://api.example.com/data", { redirect: "manual" })
.then(response => {
if (response.status === 301 || response.status === 302) {
const newLocation = response.headers.get("Location");
return fetch(newLocation, { credentials: "include" });
}
})
.then(data => console.log(data))
.catch(error => console.error(error));
2. Configuring the Backend to Allow CORS on Redirected Responses
Ensure both the original and redirected responses include properCORS headers.
Express.js Example:
const express = require("express");
const cors = require("cors");
const app = express();
app.use(cors({
origin: "https://yourfrontend.com",
credentials: true
}));
app.get("/redirect", (req, res) => {
res.redirect("https://another-origin.com/data");
});
app.listen(3000, () => console.log("Server running on port 3000"));
Ensure that https://another-origin.com/data
also includes the required CORS headers.
Example: Fixing CORS Redirect Issues in an Express Server
Problem: Redirect Without CORS Headers
app.get("/old-endpoint", (req, res) => {
res.redirect("https://new-api.example.com/data");
});
If https://new-api.example.com/data
does not return CORS headers, the browser will block the request.
Solution: Add CORS Headers to Redirects
app.get("/old-endpoint", (req, res) => {
res.setHeader("Access-Control-Allow-Origin", "https://yourfrontend.com");
res.setHeader("Access-Control-Allow-Credentials", "true");
res.redirect("https://new-api.example.com/data");
});
Backend Fix on https://new-api.example.com/data
app.use((req, res, next) => {
res.setHeader("Access-Control-Allow-Origin", "https://yourfrontend.com");
res.setHeader("Access-Control-Allow-Credentials", "true");
next();
});
Debugging CORS and Redirects
-
Check the Network Tab in Developer Tools
- Look at the Request URL and Response Headers.
- Check if the redirected response includes
Access-Control-Allow-Origin
-
Use
redirect: "manual"
in Fetch API- This helps identify where the redirection is happening.
-
Inspect Browser Console Errors
- The error message will indicate which request was blocked.
Conclusion
CORS blocks redirects when the redirected response lacks proper CORS headers. To fix this, ensure both the original and redirected responses include: Access-Control-Allow-Origin
Key solutions include:
- Handling redirects manually in the frontend (fetch API)
redirect: "manual"
- Configuring the backend to return CORS headers for all responses, including redirects.
- Debugging with browser dev tools to inspect network requests and response headers.
By applying these fixes, you can prevent CORS-related redirect issues and enable smooth cross-origin requests.