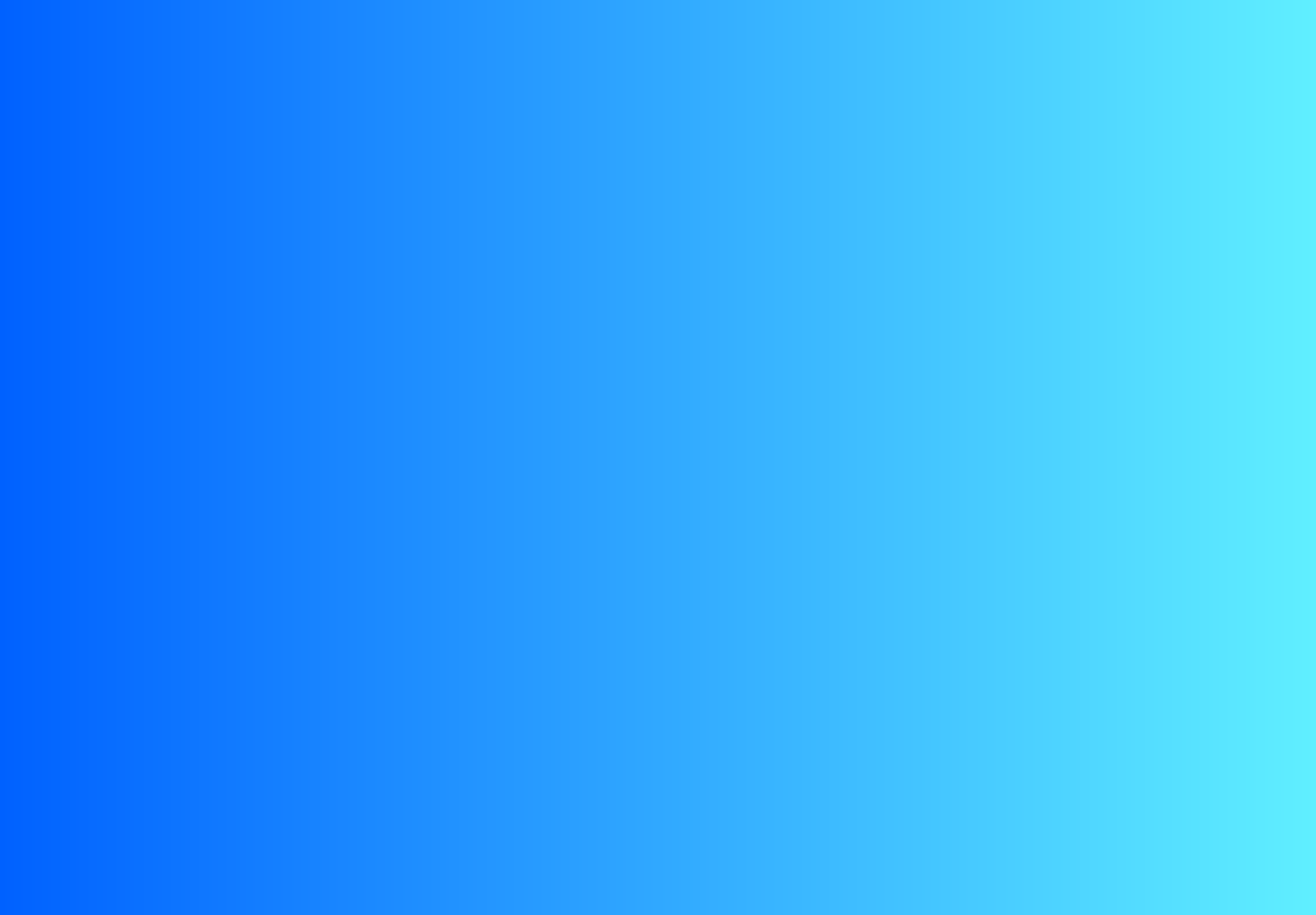
Credentials Requests Not Allowed
This error occurs when a frontend request includes credentials (such as cookies or authentication headers), but the server does not allow credentialed requests through its CORS policy.
When does this error occur?
You might see this error in the browser console when making a fetch or XMLHttpRequest request with credentials. Example:
fetch("https://api.example.com/data", {
method: "GET",
credentials: "include",
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
If the server does not explicitly allow credentials in its CORS headers, the browser will block the request.
How Credentials Work in CORS
credentials: ‘include’ in Fetch Requests
In the Fetch API, the credentials option determines whether cookies and authentication headers should be sent with cross-origin requests. It has three values:
"omit"
(default) – No credentials are sent."same-origin"
– Credentials are sent only for same-origin requests."include"
– Credentials are sent in all requests, even to other origins.
Example:
fetch("https://example.com/protected", {
method: "GET",
credentials: "include",
});
You can test your API for credentials support with CORS-Testing Tool.
withCredentials in XMLHttpRequest
For older XMLHttpRequest:
const xhr = new XMLHttpRequest();
xhr.open("GET", "https://example.com/protected");
xhr.withCredentials = true;
xhr.send();
Cookies and Authorization Headers in Cross-Origin Requests
Browsers block cross-origin credentialed requests unless the server explicitly allows them. This applies to:
- Cookies
document.cookie
- Authorization headers
Authorization: Bearer <token>
Common Causes of the Error
1. The Server Does Not Allow Credentials in CORS
By default, most servers block credentialed cross-origin requests for security reasons.
2. Missing Access-Control-Allow-Credentials Header
If the server does not include the Access-Control-Allow-Credentials: true
header, the browser will block the request.
3. The Access-Control-Allow-Origin Header is Set to *
A common mistake is using:
Access-Control-Allow-Origin: *
When credentials are involved, *
is not allowed. The server must specify a specific origin.
Fixing the Issue
1. Configuring the Server to Allow Credentials
Your backend must include the following headers:
Access-Control-Allow-Origin: https://yourfrontend.com
Access-Control-Allow-Credentials: true
Example in Express.js:
app.use((req, res, next) => {
res.header("Access-Control-Allow-Origin", "https://yourfrontend.com");
res.header("Access-Control-Allow-Credentials", "true");
res.header("Access-Control-Allow-Methods", "GET, POST, PUT, DELETE");
next();
});
2. Setting Proper CORS Headers
Wrong (Credentials will be blocked):
Access-Control-Allow-Origin: *
Access-Control-Allow-Credentials: true
Correct:
Access-Control-Allow-Origin: https://yourfrontend.com
Access-Control-Allow-Credentials: true
3. Handling Credentials in the Frontend
Ensure your frontend is making requests with credentials: "include"
Example: Fixing Credentials Issue in an Express Server
const express = require("express");
const cors = require("cors");
const app = express();
const corsOptions = {
origin: "https://yourfrontend.com",
credentials: true,
};
app.use(cors(corsOptions));
app.get("/protected", (req, res) => {
res.json({ message: "This is a protected resource" });
});
app.listen(3000, () => console.log("Server running on port 3000"));
Debugging CORS Credentials Issues
-
Check Network Requests (Chrome DevTools > Network > Headers)
- Ensure the request contains
credentials: include
- Verify Access-Control-Allow-Credentials in the response headers.
Access-Control-Allow-Credentials: true
- Ensure the request contains
-
Look for Console Errors
"Credentials requests not allowed"
usually means a misconfigured backend.
-
Check Server Logs
- Ensure your backend is setting the correct CORS headers.
Conclusion
The “Credentials Requests Not Allowed” error occurs when a request with credentials is made to a server that doesn’t explicitly allow them.
credentials: "include"
To fix this:
- Ensure the server includes
Access-Control-Allow-Credentials: true
in its response. - The Access-Control-Allow-Origin header must not be * — use a specific origin instead.
Access-Control-Allow-Origin: https://someorigin.com
- Debug using browser dev tools to check request headers and response headers.
By correctly configuring CORS headers, you can enable credentialed requests while maintaining security.