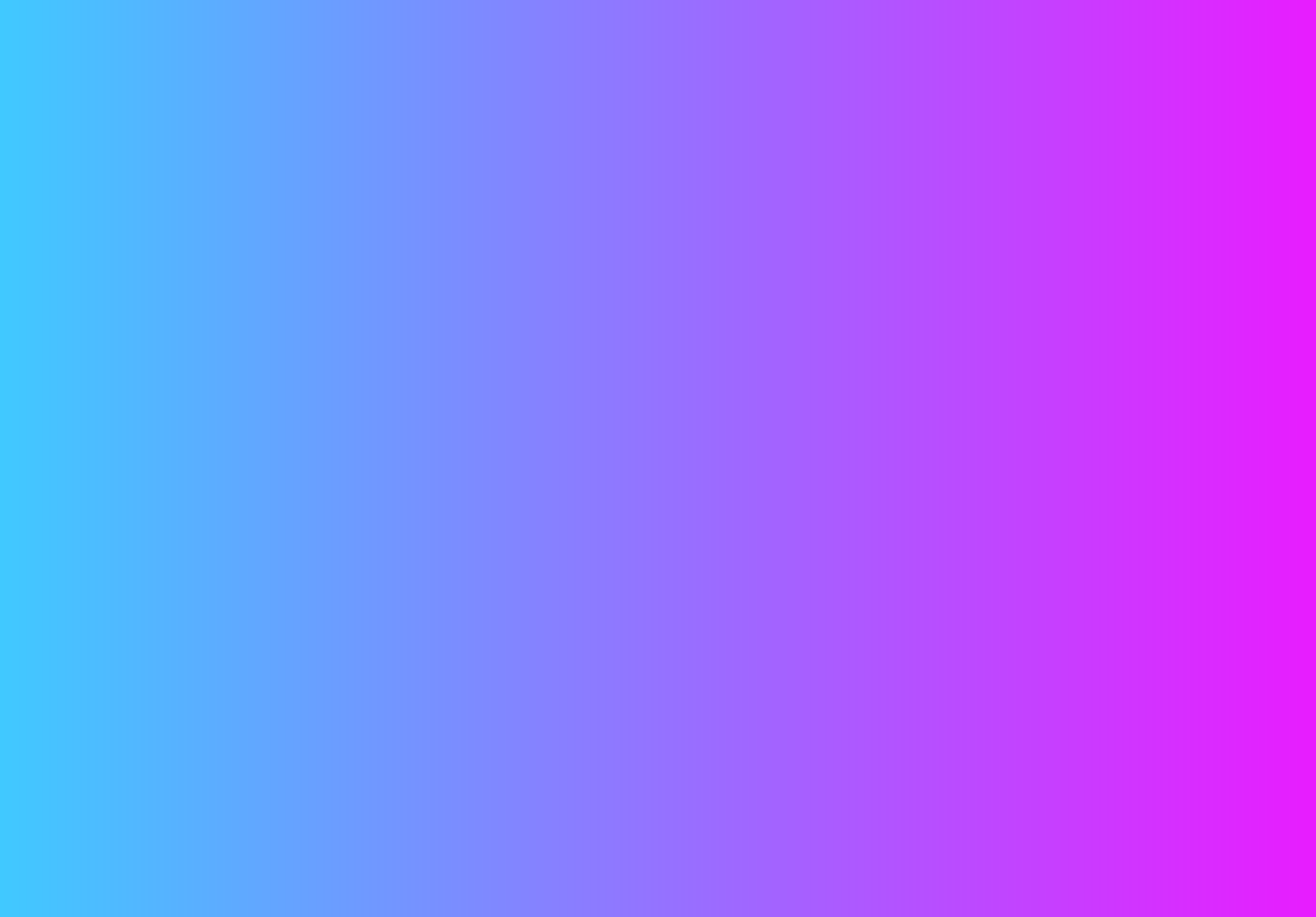
Invalid Access-Control-Allow-Origin Value
The Invalid Access-Control-Allow-Origin Value error occurs when the server responds with an incorrect or malformed value for the Access-Control-Allow-Origin header. This header is critical for enabling cross-origin requests, and if its value is invalid, the browser will block the request. Let’s dive into why this happens, how to fix it.
Why Does This Error Occur?
The Access-Control-Allow-Origin header specifies which origins are allowed to access a resource. If the value of this header is invalid, the browser will reject the response. Common causes of this error include:
- Incorrect Format: The header value must be a valid origin e.g.
https://example.com
or*
(to allow all origins). - Multiple Values: The header should only contain a single origin or
*
Multiple origins are not allowed. - Mismatched Origin: If the header specifies a specific origin e.g.
https://frontend.com
but the request comes from a different origin e.g.https://example.com
the browser will block the request.
How to Fix the Error
1. Ensure the Header Value is Valid
The Access-Control-Allow-Origin header must have a valid value. Here are the correct formats:
- Allow all origins:
Access-Control-Allow-Origin: *
- Allow a specific origin:
Access-Control-Allow-Origin: https://example.com
Example in Node.js (Express):
app.use((req, res, next) => {
const allowedOrigins = ['https://example.com', 'https://frontend.com'];
const origin = req.headers.origin;
if (allowedOrigins.includes(origin)) {
res.header('Access-Control-Allow-Origin', origin); // Allow specific origin
}
res.header('Access-Control-Allow-Methods', 'GET, POST, PUT, DELETE');
res.header('Access-Control-Allow-Headers', 'Content-Type, Authorization');
next();
});
Example in Python (Flask):
from flask import Flask, request, jsonify
from flask_cors import CORS
app = Flask(__name__)
@app.after_request
def add_cors_headers(response):
allowed_origins = ['https://example.com', 'https://frontend.com']
origin = request.headers.get('Origin')
if origin in allowed_origins:
response.headers['Access-Control-Allow-Origin'] = origin
response.headers['Access-Control-Allow-Methods'] = 'GET, POST, PUT, DELETE'
response.headers['Access-Control-Allow-Headers'] = 'Content-Type, Authorization'
return response
@app.route('/api/data')
def get_data():
return jsonify(message="Hello, CORS is enabled!")
if __name__ == '__main__':
app.run(port=3000)
Example in Nginx:
If you’re using Nginx, ensure the add_header directive is correctly configured:
location /api/ {
if ($http_origin ~* (https://example.com|https://frontend.com)) {
add_header 'Access-Control-Allow-Origin' '$http_origin';
}
add_header 'Access-Control-Allow-Methods' 'GET, POST, PUT, DELETE';
add_header 'Access-Control-Allow-Headers' 'Content-Type, Authorization';
proxy_pass http://backend-server;
}
2. Avoid Multiple Values
The Access-Control-Allow-Origin header can only have one value. If you try to include multiple origins, the browser will reject the response. For example, the following is invalid:
Access-Control-Allow-Origin: https://example.com, https://frontend.com
Instead, dynamically set the header based on the request origin, as shown in the examples above.
3. Handle Preflight Requests
If your request includes custom headers or non-standard methods, the browser will send a preflight request (OPTIONS). Ensure your server responds with the correct Access-Control-Allow-Origin header in the preflight response.
Example Preflight Response:
HTTP/1.1 204 No Content
Access-Control-Allow-Origin: https://example.com
Access-Control-Allow-Methods: GET, POST, PUT, DELETE
Access-Control-Allow-Headers: Content-Type, Authorization
Best Practices for CORS Implementation
- Validate Header Values: Ensure the Access-Control-Allow-Origin header has a valid value (either * or a specific origin).
- Avoid Multiple Origins: The header should only contain one origin or *.
- Handle Preflight Requests: Ensure your server correctly responds to OPTIONS requests.
Conclusion
The Invalid Access-Control-Allow-Origin Value error usually stems from misconfigured CORS policies, such as improperly set headers or conflicting origin rules. To resolve this, ensure the server correctly specifies allowed origins, avoids wildcard (*
) with credentials, and dynamically sets the header when needed. Proper debugging and adherence to security best practices can help prevent this issue.