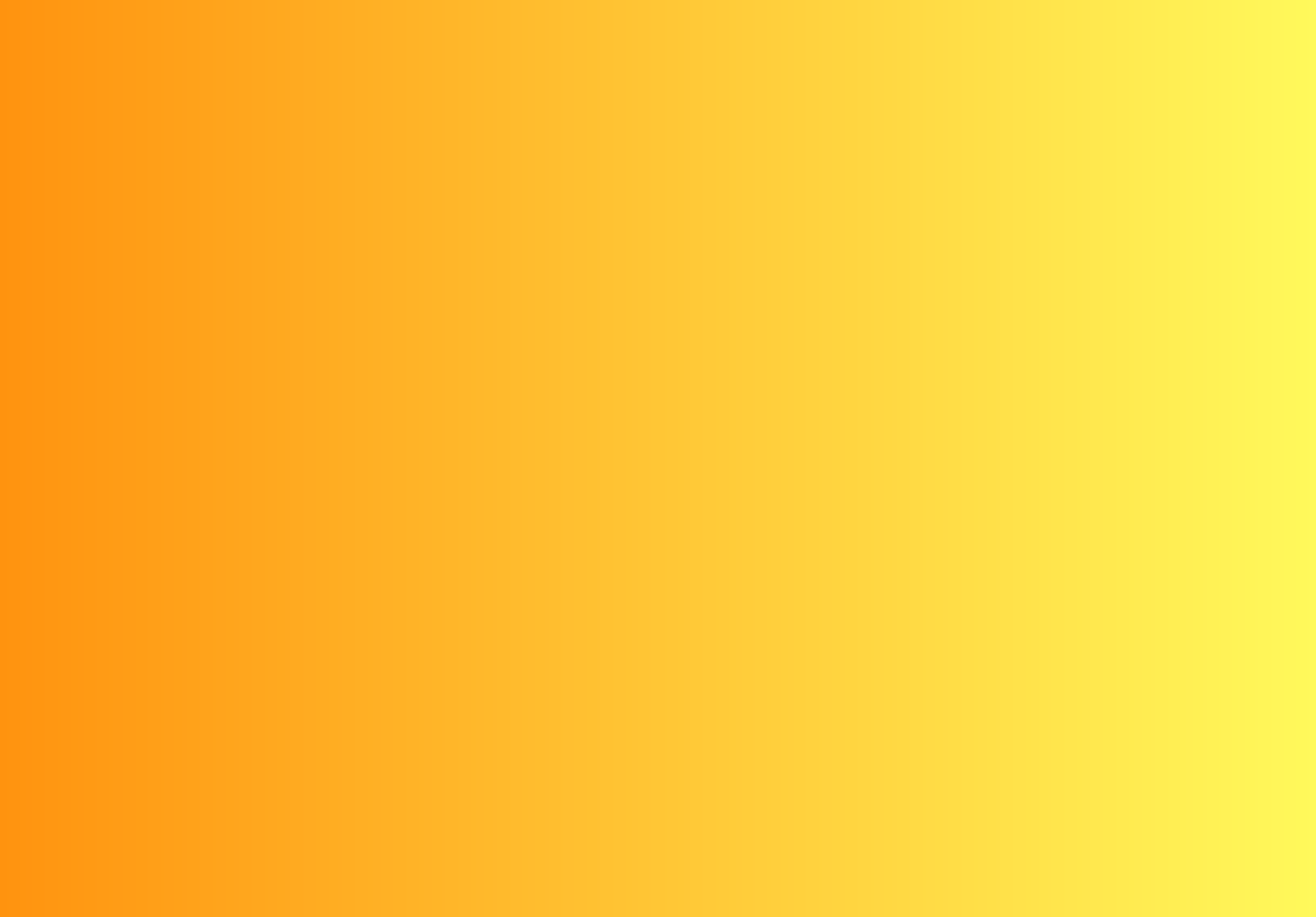
Method Not Allowed by CORS
What Does “Method Not Allowed by CORS” Mean?
The “Method Not Allowed by CORS” error occurs when a client (browser or API consumer) sends an HTTP request using a method e.g.: [POST, PUT, DELETE]
that is not permitted by the server’s CORS policy. This typically happens when:
- The server does not explicitly allow the HTTP method being used in the request.
- The server does not return the
Access-Control-Allow-Methods
header. - A preflight request
OPTIONS
method is rejected by the server.
Understanding Preflight Requests
For non-simple HTTP requests e.g., requests with [PUT, DELETE]
or custom headers like Authorization
the browser sends a preflight request before the actual request.
A preflight request is an OPTIONS
request sent by the browser to check:
- Whether the requested method
[PUT, DELETE]
etc. is allowed by the server. - If required headers
Authorization
Content-Type
etc. are permitted.
If the server does not include the required CORS headers in the OPTIONS
response, the actual request is blocked.
Common Causes & Fixes
1. The Server Does Not Allow the HTTP Method
Issue:
The server is not configured to accept the HTTP method e.g.: `[PUT, DELETE, PATCH].
Fix:
Ensure the server includes the Access-Control-Allow-Methods
header in the response:
Access-Control-Allow-Methods: GET, POST, PUT, DELETE, OPTIONS
This tells the browser that these HTTP methods are allowed for cross-origin requests.
For Express.js (Node.js):
app.use((req, res, next) => {
res.header("Access-Control-Allow-Methods", "GET, POST, PUT, DELETE, OPTIONS");
next();
});
For Flask (Python):
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.after_request
def add_cors_headers(response):
response.headers['Access-Control-Allow-Methods'] = 'GET, POST, PUT, DELETE, OPTIONS'
return response
2. The Server Does Not Respond to Preflight Requests
Issue:
If the server does not handle OPTIONS
requests properly, the browser blocks the actual request.
Fix:
Ensure the server explicitly handles preflight OPTIONS
requests.
For Express.js:
app.options("*", (req, res) => {
res.header("Access-Control-Allow-Methods", "GET, POST, PUT, DELETE, OPTIONS");
res.send();
});
For Flask:
@app.route('/your-endpoint', methods=['OPTIONS'])
def handle_options():
response = jsonify()
response.headers.add("Access-Control-Allow-Methods", "GET, POST, PUT, DELETE, OPTIONS")
return response
3. The Request Method is Restricted by the API or CDN
Issue:
Some APIs (e.g., third-party APIs, AWS API Gateway) only allow specific HTTP methods, blocking others.
Fix:
Check the API documentation and see if it supports the requested method. You may need to:
- Use an API key to access certain methods.
- Change the request method e.g.:
PATCH
instead of:PUT
- Modify the API Gateway configuration (if using AWS).
Conclusion
The Method Not Allowed by CORS error occurs when the server does not include the requested HTTP method in the Access-Control-Allow-Methods
header during the preflight response. To fix this, ensure the server explicitly allows the required methods and correctly handles preflight requests. Proper server-side configuration and debugging can help prevent this issue.