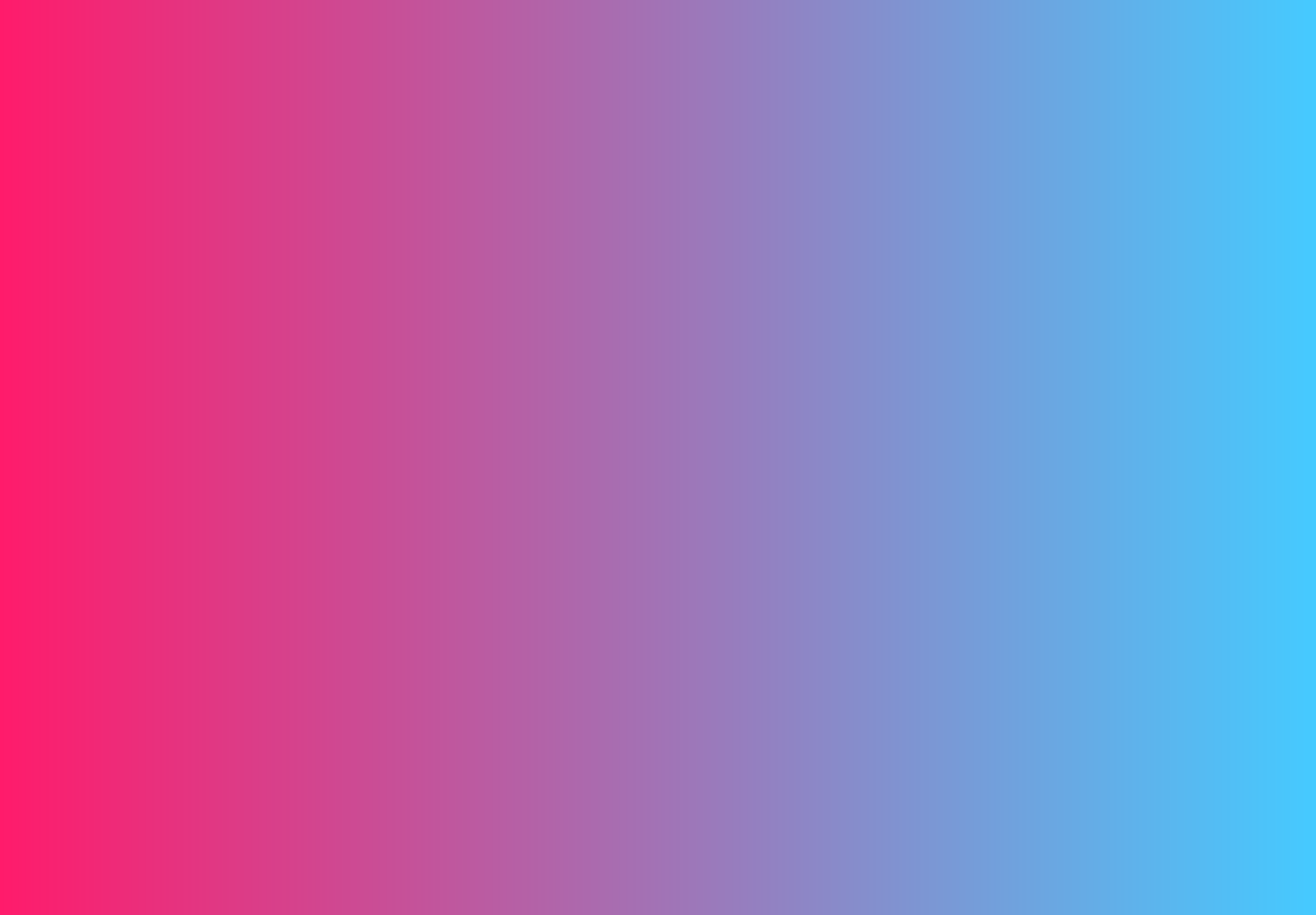
No Access-Control-Allow-Origin Header
When working with APIs, you might encounter the error: “No Access-Control-Allow-Origin Header”. This error occurs when a web application running in a browser tries to make a request to a server on a different origin (domain, protocol, or port), and the server doesn’t include the necessary CORS headers in its response. In this guide, we’ll explore why this error happens, how to fix it.
Why Does This Error Occur?
Browsers enforce the Same-Origin Policy for security reasons. This policy prevents a web page from making requests to a different domain than the one that served the web page. However, there are legitimate cases where you need to make cross-origin requests, such as when your frontend hosted on: https://example.com
needs to communicate with a backend API hosted on: https://api.example.com
To allow cross-origin requests, the server must include the Access-Control-Allow-Origin
header in its response. If this header is missing, the browser blocks the request, and you’ll see the error: “No Access-Control-Allow-Origin Header”.
How to Fix the Error
1. Server-Side Solution: Set the Access-Control-Allow-Origin Header
The most straightforward way to resolve this error is to configure your server to include the Access-Control-Allow-Origin
header in its responses. Here’s how you can do it for different server environments:
Example in Node.js (Express):
const express = require('express');
const app = express();
app.use((req, res, next) => {
res.header('Access-Control-Allow-Origin', '*'); // Allow all origins
res.header('Access-Control-Allow-Methods', 'GET, POST, PUT, DELETE');
res.header('Access-Control-Allow-Headers', 'Content-Type, Authorization');
next();
});
app.get('/api/data', (req, res) => {
res.json({ message: 'Hello, CORS is enabled!' });
});
app.listen(3000, () => {
console.log('Server running on http://localhost:3000');
});
example in node.js using cors
const express = require('express');
const app = express();
const cors = require('cors');
app.use(cors());
app.get('/api/data', (req, res) => {
res.json({ message: 'Hello, CORS is enabled!' });
});
app.listen(3000, () => {
console.log('Server running on http://localhost:3000');
});
example in python (flask):
from flask import flask, jsonify
from flask_cors import cors
app = flask(__name__)
cors(app) # enable cors for all routes
@app.route('/api/data')
def get_data():
return jsonify(message="hello, cors is enabled!")
if __name__ == '__main__':
app.run(port=3000)
example in nginx:
if you’re using nginx as a reverse proxy, you can add the following to your configuration:
location /api/ {
add_header 'access-control-allow-origin' '*';
add_header 'access-control-allow-methods' 'get, post, put, delete';
add_header 'access-control-allow-headers' 'content-type, authorization';
proxy_pass http://backend-server;
}
2. handling preflight requests
for certain types of requests (e.g., those with custom headers or non-standard methods), the browser sends a preflight request (an options request) options
to check if the server allows the actual request. your server must respond to preflight requests with the appropriate CORS headers.
example preflight response:
http/1.1 204 no content
access-control-allow-origin: *
access-control-allow-methods: get, post, put, delete
access-control-allow-headers: content-type, authorization
3. client-side workarounds
if you don’t have control over the server, you can use client-side workarounds. however, these are not recommended for production environments due to security and performance concerns.
using a proxy server:
you can set up a proxy server to forward requests from your frontend to the backend. the proxy server adds the necessary CORS headers to the response.
best practices for cors implementation
- restrict allowed origins: instead of using wildcard (*) , specify the exact origins that are allowed to access your api. for example:
cors({origin:['https://frontend.com']})
- use secure methods and headers: only allow necessary http methods and headers in the headers.
access-control-allow-methods access-control-allow-headers
- handle preflight requests: ensure your server correctly responds to
options
requests.
Conclusion
The No Access-Control-Allow-Origin Header error occurs when the server does not include the Access-Control-Allow-Origin
header in its response, preventing the browser from allowing cross-origin requests. To fix this, ensure the server explicitly sets this header with an allowed origin or *
if appropriate. Proper server-side configuration is key to resolving this issue.