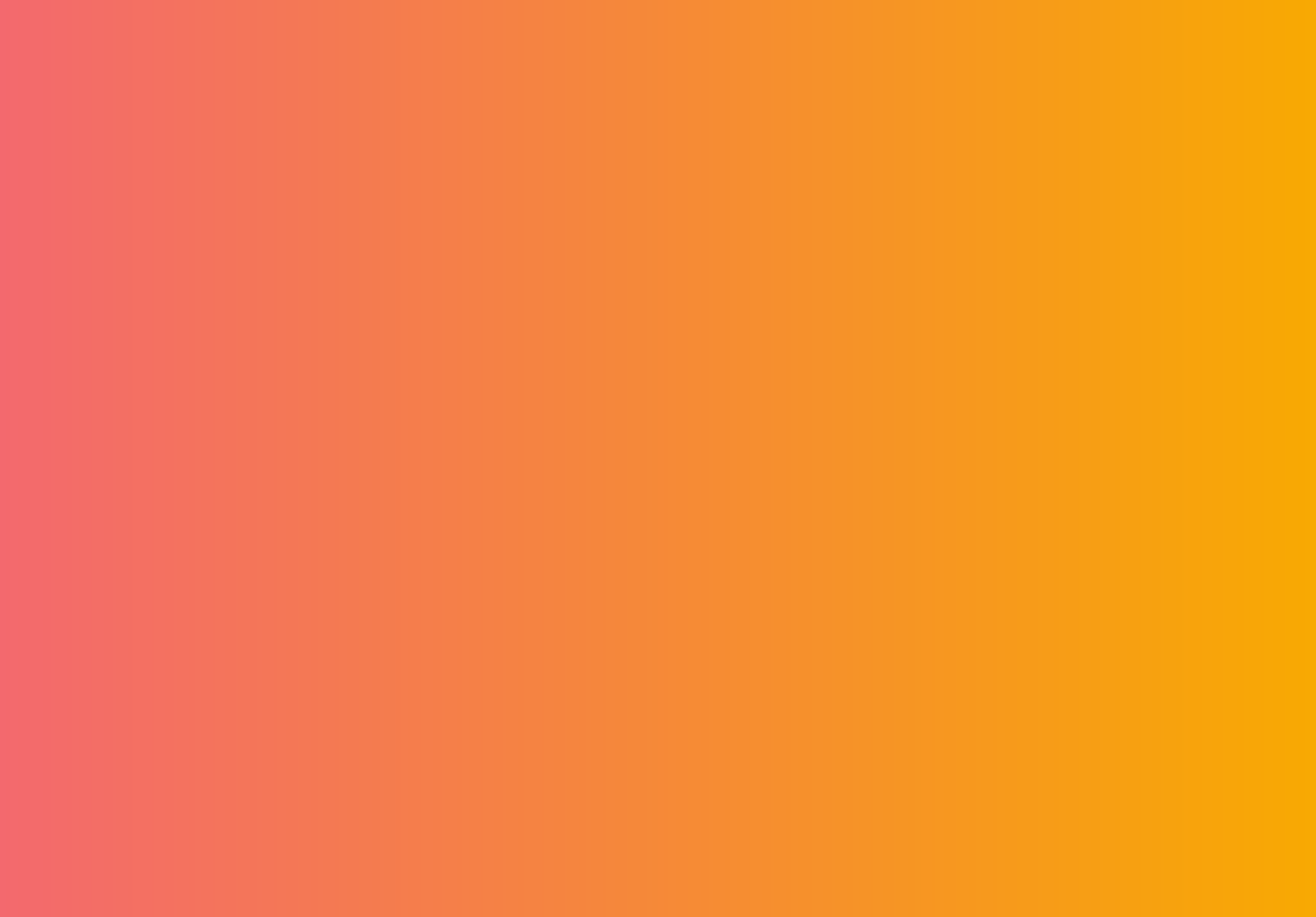
Origin Mismatch
An Origin Mismatch error in CORS happens when the browser detects that the request’s Origin
header does not match the allowed origins defined by the server. Here’s how you can debug and fix it:
Possible Causes & Fixes
1. Check the Origin
Header in the Request
Before anything, open the Network tab in your browser’s Developer Tools and inspect the request headers. You’ll see something like this:
Origin: http://localhost:3000
- If your frontend is running on
http://localhost:3000
, but your backend only allowshttp://localhost:3001
, it will reject the request. - To fix this, update your CORS configuration to include the correct origin.
2. Fix CORS Configuration in the Backend
Right now, you have:
cors({ origin: ['*'] })
But origin: ['*']
is not valid in CORS middleware. Instead, use:
const cors = require('cors');
const express = require('express');
const app = express();
app.use(cors({
origin: '*', // Allow all origins
methods: ['GET', 'POST', 'OPTIONS'],
allowedHeaders: ['Content-Type', 'Authorization']
}));
app.use(express.json());
Alternatively, to allow only your frontend:
app.use(cors({
origin: 'http://localhost:3000', // Allow requests only from your frontend
methods: ['GET', 'POST', 'OPTIONS'],
allowedHeaders: ['Content-Type', 'Authorization']
}));
3. Allow Credentials if Needed
If your API requires credentials (cookies, sessions), update both frontend and backend:
Backend:
app.use(cors({
origin: 'http://localhost:3000',
credentials: true
}));
Frontend:
fetch('http://localhost:3001/cors/validator', {
method: 'POST',
credentials: 'include', // Send cookies if required
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify(payload)
});
4. Preflight Requests (OPTIONS Method)
Sometimes browsers send an OPTIONS request (preflight) before the actual request. Make sure your backend handles it:
app.options('*', cors());
Conclusion
The Origin Mismatch error occurs when the Origin
in the request does not match the allowed origins specified by the server in the Access-Control-Allow-Origin
header. This often happens when attempting requests from unauthorized domains. To resolve this, ensure that the server correctly includes the expected origin in its response or adjusts CORS settings to allow the necessary domains while maintaining security.